A simple implementation of stale-while-revalidate caching using async/await
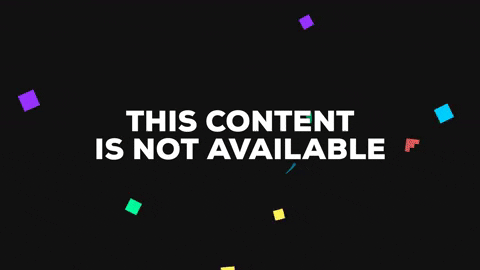
New is not always better! Returning cached responses can improve app speed and resilience. Keep reading to find out why and how it works.
Recently I faced the problem to write a service worker that implements a very basic stale-while-revalidate caching strategy. Searching through the web there are many proposed implementations of this pattern, but I found none that uses state-of-the-art, low overhead async/await. Personally I cannot stand looking at 3+ nested .then
statements, especially as async functions are supported by all relevant browsers in 2021. For this post I consciously formatted the code in the most readable way. Of course there are some opportunities to minify. Here is the code:
1 | async function fetchAndCacheIfOk(event) { |
The implementation satisfies the tree core requirements I needed to meet:
- Intercepts fetch requests and instantly serves a cached response if one is available
- Requests and a fresh response every time it intercepts fetch and saves it to the CacheStorage
- Does not cache non-ok responses (everything that is 200-299, feel free to change this if it does not suit your needs)
The main benefits of this strategy and also the reason why I chose it is that it increases resilience of the application with regard to some vital endpoints occasionally not being available. In my specific case it is used to cache requests that are needed on app-init and that would cause the init process to fail if unavailable. A pre-caching strategy is not possible it this specific scenario because the requests contain authentication and can vary from user to user.
As a side effect, the responses of the cached requests are served almost instantaneous. In my case this decreased the average startup time of the app a bit, which is nice.
Feel free to use, extend or improve this basic implementation in any of your projects!